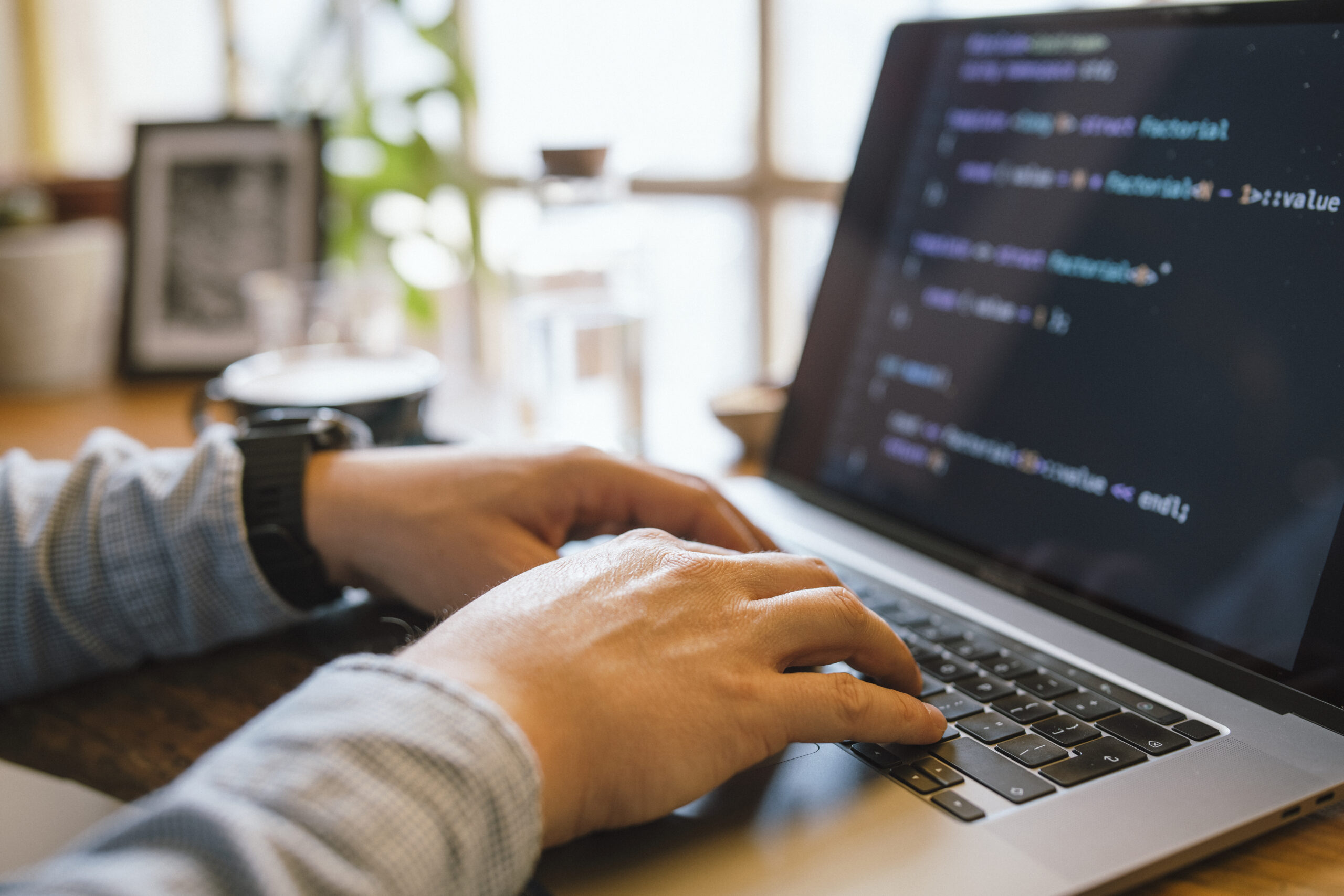
Debugging is one of the most essential — still frequently neglected — competencies inside a developer’s toolkit. It isn't really pretty much correcting damaged code; it’s about knowing how and why issues go Completely wrong, and learning to Believe methodically to solve troubles successfully. Whether or not you're a newbie or possibly a seasoned developer, sharpening your debugging capabilities can help save hrs of annoyance and radically help your efficiency. Here are several strategies to help builders amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Equipment
One of several quickest methods builders can elevate their debugging techniques is by mastering the instruments they use every single day. Although producing code is a single Portion of improvement, knowing ways to communicate with it efficiently throughout execution is Similarly significant. Modern day improvement environments occur Outfitted with effective debugging abilities — but quite a few developers only scratch the area of what these instruments can do.
Take, one example is, an Integrated Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools allow you to established breakpoints, inspect the value of variables at runtime, move by code line by line, and in some cases modify code around the fly. When made use of accurately, they let you notice just how your code behaves for the duration of execution, that is a must have for monitoring down elusive bugs.
Browser developer tools, which include Chrome DevTools, are indispensable for front-end developers. They allow you to inspect the DOM, observe network requests, perspective true-time efficiency metrics, and debug JavaScript in the browser. Mastering the console, resources, and network tabs can turn discouraging UI concerns into workable duties.
For backend or procedure-degree builders, resources like GDB (GNU Debugger), Valgrind, or LLDB present deep Handle above functioning processes and memory administration. Understanding these applications might have a steeper Mastering curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Beyond your IDE or debugger, turn into snug with Edition Regulate methods like Git to grasp code heritage, locate the exact minute bugs were being introduced, and isolate problematic adjustments.
In the long run, mastering your tools implies heading outside of default configurations and shortcuts — it’s about building an intimate familiarity with your progress environment to ensure that when troubles crop up, you’re not shed at the hours of darkness. The better you realize your resources, the more time it is possible to shell out fixing the actual issue in lieu of fumbling by the method.
Reproduce the challenge
Among the most significant — and often ignored — measures in successful debugging is reproducing the challenge. Just before jumping into the code or earning guesses, builders need to have to make a constant environment or state of affairs wherever the bug reliably appears. With out reproducibility, correcting a bug will become a match of opportunity, typically leading to squandered time and fragile code variations.
Step one in reproducing an issue is accumulating as much context as possible. Talk to issues like: What actions triggered The problem? Which environment was it in — progress, staging, or creation? Are there any logs, screenshots, or error messages? The greater detail you may have, the less complicated it gets to be to isolate the precise circumstances less than which the bug happens.
As you’ve collected more than enough data, attempt to recreate the situation in your local natural environment. This could necessarily mean inputting precisely the same data, simulating related user interactions, or mimicking process states. If the issue appears intermittently, take into consideration creating automatic tests that replicate the edge scenarios or state transitions concerned. These checks not just assistance expose the issue and also prevent regressions Sooner or later.
In some cases, the issue could possibly be environment-certain — it would materialize only on specific functioning methods, browsers, or beneath individual configurations. Utilizing instruments like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this sort of bugs.
Reproducing the situation isn’t just a stage — it’s a frame of mind. It involves persistence, observation, plus a methodical solution. But once you can constantly recreate the bug, you are previously midway to correcting it. Which has a reproducible state of affairs, You should use your debugging resources a lot more properly, take a look at probable fixes safely and securely, and converse additional clearly with all your team or users. It turns an abstract complaint into a concrete challenge — and that’s exactly where developers thrive.
Read and Understand the Mistake Messages
Mistake messages will often be the most beneficial clues a developer has when something goes wrong. Rather than seeing them as frustrating interruptions, builders should really master to take care of error messages as direct communications in the system. They often show you just what exactly took place, the place it occurred, and occasionally even why it transpired — if you understand how to interpret them.
Commence by reading through the message carefully As well as in entire. Numerous builders, specially when beneath time stress, glance at the main line and promptly start off producing assumptions. But deeper within the mistake stack or logs may well lie the real root result in. Don’t just copy and paste mistake messages into search engines like google and yahoo — examine and recognize them initially.
Split the mistake down into sections. Is it a syntax mistake, a runtime exception, or even a logic mistake? Does it point to a certain file and line quantity? What module or functionality induced it? These questions can tutorial your investigation and stage you towards the accountable code.
It’s also practical to grasp the terminology from the programming language or framework you’re working with. Error messages in languages like Python, JavaScript, or Java normally stick to predictable styles, and Studying to acknowledge these can drastically accelerate your debugging process.
Some glitches are imprecise or generic, and in Those people circumstances, it’s very important to examine the context through which the error transpired. Look at associated log entries, enter values, and up to date adjustments from the codebase.
Don’t overlook compiler or linter warnings either. These typically precede larger sized issues and provide hints about probable bugs.
Ultimately, error messages are usually not your enemies—they’re your guides. Understanding to interpret them effectively turns chaos into clarity, helping you pinpoint concerns speedier, cut down debugging time, and turn into a much more productive and self-assured developer.
Use Logging Sensibly
Logging is Probably the most effective equipment in the developer’s debugging toolkit. When made use of correctly, it offers real-time insights into how an application behaves, aiding you fully grasp what’s going on underneath the hood while not having to pause execution or action in the code line by line.
A very good logging strategy starts with realizing what to log and at what stage. Prevalent logging degrees involve DEBUG, Facts, WARN, ERROR, and FATAL. Use DEBUG for comprehensive diagnostic info in the course of improvement, Information for general occasions (like effective start-ups), Alert for probable troubles that don’t split the application, ERROR for actual problems, and Lethal in the event the process can’t continue.
Steer clear of flooding your logs with abnormal or irrelevant knowledge. An excessive amount logging can obscure critical messages and slow down your method. Concentrate on key situations, point out alterations, input/output values, and important conclusion details with your code.
Format your log messages clearly and continually. Include things like context, including timestamps, request IDs, and performance names, so it’s simpler to trace concerns in dispersed programs or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs here programmatically.
Through debugging, logs Allow you to keep track of how variables evolve, what problems are met, and what branches of logic are executed—all without the need of halting the program. They’re Primarily important in creation environments where stepping by code isn’t achievable.
On top of that, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
Finally, smart logging is about equilibrium and clarity. Using a perfectly-imagined-out logging solution, you are able to decrease the time it takes to spot difficulties, acquire further visibility into your purposes, and improve the Total maintainability and trustworthiness of one's code.
Consider Similar to a Detective
Debugging is not just a specialized undertaking—it is a form of investigation. To efficiently establish and fix bugs, developers need to method the method similar to a detective resolving a mystery. This attitude will help stop working elaborate issues into manageable elements and comply with clues logically to uncover the foundation induce.
Start by gathering evidence. Look at the signs of the challenge: mistake messages, incorrect output, or general performance issues. Just like a detective surveys a crime scene, collect as much related details as you'll be able to with no jumping to conclusions. Use logs, examination situations, and consumer reviews to piece collectively a clear picture of what’s happening.
Next, form hypotheses. Ask you: What can be producing this behavior? Have any changes recently been built into the codebase? Has this challenge transpired just before below similar instances? The target is usually to slim down choices and identify opportunity culprits.
Then, take a look at your theories systematically. Try and recreate the trouble inside a managed setting. Should you suspect a specific purpose or element, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, inquire your code thoughts and Permit the outcome lead you nearer to the truth.
Pay near interest to small aspects. Bugs typically hide during the minimum anticipated sites—similar to a missing semicolon, an off-by-a person error, or simply a race issue. Be thorough and individual, resisting the urge to patch the issue devoid of completely comprehending it. Non permanent fixes could disguise the real trouble, only for it to resurface later on.
And finally, keep notes on Whatever you tried and figured out. Just as detectives log their investigations, documenting your debugging approach can save time for foreseeable future challenges and aid Many others realize your reasoning.
By imagining like a detective, developers can sharpen their analytical techniques, approach difficulties methodically, and develop into more effective at uncovering hidden difficulties in complex techniques.
Generate Tests
Producing checks is one of the best solutions to improve your debugging expertise and Total progress performance. Tests not just aid capture bugs early but will also function a safety net that gives you self-assurance when generating improvements towards your codebase. A well-tested application is simpler to debug as it means that you can pinpoint accurately where and when a problem occurs.
Start with device checks, which deal with individual capabilities or modules. These compact, isolated checks can immediately expose irrespective of whether a selected bit of logic is Performing as predicted. Each time a examination fails, you right away know in which to search, substantially decreasing the time spent debugging. Device checks are Specially valuable for catching regression bugs—concerns that reappear following previously remaining fastened.
Following, integrate integration tests and close-to-conclusion exams into your workflow. These assist ensure that many portions of your application work alongside one another efficiently. They’re especially useful for catching bugs that come about in sophisticated methods with various elements or solutions interacting. If a little something breaks, your assessments can tell you which Component of the pipeline failed and less than what situations.
Writing assessments also forces you to Assume critically about your code. To check a function properly, you need to be aware of its inputs, expected outputs, and edge conditions. This amount of understanding In a natural way leads to higher code composition and fewer bugs.
When debugging a concern, writing a failing examination that reproduces the bug can be a strong starting point. Once the examination fails consistently, it is possible to deal with fixing the bug and look at your exam pass when The problem is fixed. This method makes sure that a similar bug doesn’t return in the future.
In a nutshell, crafting tests turns debugging from a annoying guessing activity into a structured and predictable method—serving to you capture more bugs, more quickly plus much more reliably.
Choose Breaks
When debugging a tricky concern, it’s effortless to be immersed in the condition—staring at your screen for hrs, striving Option just after solution. But Probably the most underrated debugging equipment is just stepping absent. Having breaks allows you reset your intellect, reduce aggravation, and often see the issue from a new perspective.
When you're too close to the code for too lengthy, cognitive fatigue sets in. You may begin overlooking apparent errors or misreading code that you simply wrote just hours earlier. In this point out, your Mind will become a lot less successful at dilemma-fixing. A short walk, a espresso split, as well as switching to a distinct activity for 10–quarter-hour can refresh your concentration. A lot of developers report discovering the root of a dilemma when they've taken time for you to disconnect, letting their subconscious work during the qualifications.
Breaks also support avoid burnout, Particularly during extended debugging periods. Sitting before a display, mentally trapped, is not simply unproductive but in addition draining. Stepping away allows you to return with renewed Electricity plus a clearer state of mind. You may perhaps quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
For those who’re caught, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–10 moment break. Use that time to maneuver all around, stretch, or do a thing unrelated to code. It may sense counterintuitive, Specifically less than restricted deadlines, however it essentially results in speedier and more effective debugging In the long term.
In short, getting breaks is not a sign of weak spot—it’s a smart method. It presents your brain Room to breathe, increases your perspective, and will help you steer clear of the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of solving it.
Understand From Each individual Bug
Each and every bug you face is a lot more than just a temporary setback—It can be a possibility to expand for a developer. Whether it’s a syntax error, a logic flaw, or possibly a deep architectural difficulty, each one can teach you one thing precious for those who make an effort to mirror and examine what went Mistaken.
Start out by inquiring yourself a couple of crucial inquiries when the bug is solved: What prompted it? Why did it go unnoticed? Could it have been caught earlier with better practices like unit testing, code reviews, or logging? The answers frequently reveal blind spots in your workflow or comprehending and assist you to Construct more powerful coding behavior shifting forward.
Documenting bugs can also be an excellent habit. Keep a developer journal or maintain a log in which you Take note down bugs you’ve encountered, the way you solved them, and Whatever you uncovered. With time, you’ll start to see patterns—recurring challenges or popular faults—you can proactively keep away from.
In crew environments, sharing Everything you've learned from the bug with the peers can be Primarily highly effective. No matter if it’s by way of a Slack message, a brief compose-up, or A fast know-how-sharing session, aiding Other people avoid the exact situation boosts group performance and cultivates a more powerful learning society.
A lot more importantly, viewing bugs as classes shifts your frame of mind from aggravation to curiosity. In lieu of dreading bugs, you’ll start off appreciating them as important portions of your advancement journey. After all, several of the very best builders aren't those who write great code, but those that repeatedly discover from their faults.
In the end, Just about every bug you repair provides a new layer in your talent set. So following time you squash a bug, have a moment to mirror—you’ll occur away a smarter, a lot more able developer due to it.
Conclusion
Improving upon your debugging abilities can take time, observe, and persistence — even so the payoff is large. It tends to make you a more successful, confident, and capable developer. The following time you happen to be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a chance to be improved at Everything you do.